Spanish Version
BI Web Services
Examples
You can download an example from the following link and check the following article.
You must follow the steps below in the specified order:
- Create a knowledge base version 8.0 or higher one.
- Use WSDL inspector tool to use the service, typing the URL where the services were installed.
- Consolidate the associated xpz selecting the "Do not overwrite SDTs" option.
- Create a model with Web interface.
- Specify, generate and compile all the objects.
- Call the Login Web Panel.
Creating a session
You must call the StartSession method and check out the status variable. Verify the Login Web Panel. Pseudocode example:
Event 'Login'
// Starting Session
&ws.StartSession (&userName, &userPassword, &session, &status)
If (&status.Error = 0)
// Login Succeeded
&session_str = &session.ToXml()
// Set Session Information to Web Session
&WebSession.Set ("Session", &session_str)
Else
&msg = &status.ErrorDescription
Do 'Error'
EndIf
EndEvent // 'Login'
Working with users
You must call the GetUserCollection method to bring the users collection and then bring the user information with the GetUser method. You must always check out the status variable. Verify the Users Web Panel. Pseudocode example:
Sub 'LoadUsers'
&element_str = ""
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.GetUserCollection(&session, &users, &status)
If &status.Error = 0
// No Error, Loading Users in comboUsers ComboBox
For &i=1 to &userCollection.Count
&item = &userCollection.Item(&i).Name
&comboUsers.Additem(&item, &item)
EndFor
&element_str = &userCollection.ToXml()
Else
&msg = &status.ErrorDescription
Do 'Error'
EndIf
&statusToXML = &status.ToXml()
EndSub
Sub 'LoadUser'
&element_str = ""
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.GetUser(&session, &userName, &user, &status)
If (&status.Error = 0)
// No Error, Loading User Information in variables
&userFullName = &user.FullName
&userName = &user.Name
&userNodeType = &user.NodeType
&userSupervisor = &user.IsSupervisor
Else
&msg = &status.ErrorDescription
Do 'Error'
Endif
EndSub
Working with metadatas
You must call the GetMetadataCollection method to bring the metadatas collection available for the user connected through the session. Then, open a metadata with the OpenMetadata method. You must always check out the status variable. Verify the Metadata Web Panel. Pseudocode example:
Sub 'LoadMetadatas'
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.GetMetadataCollection(&session, &metadatas, &status)
If &status.Error = 0
// No Error, Loading Metadatas in &ComboMetadatas ComboBox
For &i=1 to &metadataCollection.Count
&item = &metadataCollection.Item(&i).Name
&ComboMetadatas.Additem(&item, &item)
EndFor
Else
&msg = &status.ErrorDescription
Do 'Error'
EndIf
&statusToXML = &status.ToXml()
EndSub
Sub 'OpenMetadata'
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.OpenMetadata(&session, &metadataName, &status)
If (&status.Error = 0)
Msg( 'Metadata was opened.')
&WebSession.Set ("Metadata", &ComboMetadatas)
Else
// Could not open metadata
&msg = &status.ErrorDescription
Do 'Error'
EndIf
EndSub // 'OpenMetadata'
Working with Business Elements
You must call the GetBusinessElementCollection method to bring the open metadata Measures, Dimensions or Attributes collection available. You can bring the element information with the GetBusinessElement method. In case it is a dimension\attribute you can call the GetBusinessElementValueCollection method to bring the values collection. You must always check out the status variable; verify the BusinessElement* Web Panels. Pseudocode example:
Sub 'LoadMeasures'
&be_type = "Measure" // Setting Business Element Type
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.GetBusinessElementCollection(&session, &be_type, &becollection, &status)
If &status.Error = 0
// Loading Measures in &ComboMeasures
For &i=1 to &businessElementCollection.Count
&item = &businessElementCollection.Item(&i).Name
&ComboMeasures.Additem(&item, &item)
EndFor
Else
&msg = &status.ErrorDescription
Do 'Error'
EndIf
EndSub
Sub 'GetBusinesesElement'
&element_str = &ComboMeasures // Name of the Business Element to get
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.GetBusinessElement(&session, &be_name, &be, &status)
If &status.Error = 0
// No Error, Loading information in variables
&beName = &businessElement.Name
&beDescription = &businessElement.Description
&beCardinality = &businessElement.Cardinality
&beNodeType = &businessElement.NodeType
Else
&msg = &status.ErrorDescription
Do 'Error'
Endif
EndSub
Sub 'GetBEValues'
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.GetBusinessElementValueCollection(&session, &be_name, &valueslike, ¬like, &values, &status)
If &status.Error = 0
// No Error, loading Values in &ComboValues
For &i=1 to &values.Count
&item = &values.Item(&i).Description
&ComboValues.Additem(&item, &item)
EndFor
Else
&msg = &status.ErrorDescription
Do 'Error'
EndIf
EndSub
Working with queries
You must call the GetQueryCollection method to bring the queries collection that the user can execute. Then, you can call some of the methods for working with queries. You must always check out the status variable. Verify the Query Web Panel. Pseudocode example:
Sub 'LoadQueries'
Call(PGetSessionFromWS, &session, &status) // Get a Session from a WebSession Variable
&ws.GetQueryCollection(&session, &only_owned, &queryCollection, &status)
If &status.Error = 0
// No Error, Loading Queries in &ComboQueries
For &i=1 to &queryCollection.Count
&item = &queryCollection.Item(&i).QueryInfo.Name
&ComboQueries.Additem(&item, &item)
EndFor
Else
&msg = &status.ErrorDescription
Do 'Error'
EndIf
EndSub
Executing queries
There are two methods to execute a query:
- GetQueryData
- GetQueryHtmlData
The first one is more powerful, enabling to execute a query in different formats. You must have previously established a session with the server, opened a metadata and selected a query to be executed in addition to configuring several parameters; Verify the ExecuteQueryWithSession Web Panel.
Pseudocode example using GetQueryData:
Event 'Execute'
Call(PGetSessionFromWS , &session, &status) // Get a Session from a WebSession Variable
If &status.Error = 0
// No Error
If &queryName = ""
msg('Query not selected.')
Else
// Get Parameters
Do 'LoadParameters'
Do 'LoadServiceProperties'
// Execute Query
&ws.GetQueryData(&session , &queryName, &Parameters, &serviceProperties, &specificformat, &xmldata, &query, &status)
If &status.Error = 0
Do 'LoadQueryData'
Else
Do 'Error'
EndIf
EndIf
Else
Do 'Error'
EndIf
EndEvent
Sub 'LoadQueryData'
&queryId = &query.QueryOpenInfo.Id
Do Case
Case &queryFormat = "XmlAbstractNoFormat"
Query.Format = 0 // Text format
Query.Caption = &Specificformat
Case &queryFormat = "HtmlOWC"
// Office Web Component
Query.Format = 1 // HTML format
// When using the 'Office Web Component' control, use a TextBlock Control.
Query.Caption = &Specificformat
Case &queryFormat = "HtmlCrossBrowserControls"
// Cross-browser control Component
Query.Format = 1
Query.Caption = &Specificformat // URL returned by the queryexecution using the Cross-browser Component
OWCTable.Visible = 0
// When using the 'DevExpresst' control, use a Embedded Page Control.
EmbPageNewControl.Source = &Specificformat
Case &queryFormat = "XmlAbstractWithFormat" // Use the default XSL File distributed with GXBI Services
Query.Format = 1 // HTML format
&xslFile = PGetBIXSLFile.Udp()
&xslPath = PGetBIServicePath.Udp()
&xslPath += &xslFile
// Do the Transformation
Query.Caption = XsltApply(&specificformat, trim(&xslPath))
Otherwise
Query.Format = 0 // Text format
Query.Caption = "Unknown Format " + &queryFormat
EndCase
EndSub
Sub 'LoadServiceProperties'
// Loading "QueryDestination"
&Property = new GXBIProperty()
&Property.Name = "QueryDestination"
&Property.Value = &queryDestination
&serviceProperties.Add( &Property )
// Loading "ExecuteQuery"
&Property = new GXBIProperty()
&Property.Name = "ExecuteQuery"
&Property.Value = trim(str(&execute))
&serviceProperties.Add( &Property )
// Loading Component Version
&Property = new GXBIProperty()
&Property.Name = "Version"
&Property.Value = &Version
&serviceProperties.Add( &Property )
// Loading "QueryFormat"
Do 'CheckQueryFormat'
&Property = new GXBIProperty()
&Property.Name = "QueryFormat"
&Property.Value = &queryFormatting
&serviceProperties.Add( &Property )
// Loading "ComponentId"
&Property = new GXBIProperty()
&Property.Name = "ComponentId"
&Property.Value = "MyComponent"
&serviceProperties.Add( &Property )
EndSub
Example:
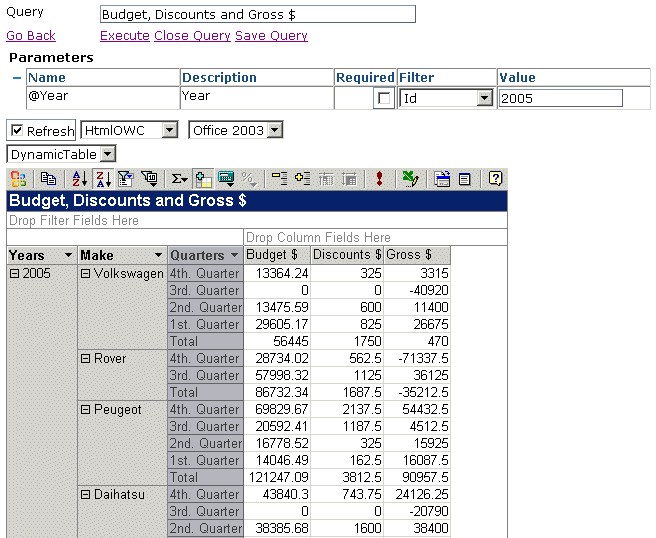
The second method (GetQueryHtmlData) enables to execute a query minimizing the quantity of parameters to be setup returning the HTML result to be embedded in any TextBlock (with HTML format) of a GeneXus Web Panel. Verify the ExecuteQuerySample Web Panel. Pseudocode example:
Do 'GetParameters' // User, Password, MetadataName, QueryName, Parameters
Do 'LoadServiceProperties' // Runtime properties in serviceProperties
// Execute the Query
&specificformat = &ws.GetQueryHtmlData(&User, &password, &metadataName, &queryName, &Parameters, &serviceProperties, &status)
If &status.Error = 0
QueryResult.Caption = &specificformat // Assign the query HTML Result to the Textblock Control
Else
Do 'Error'
EndIf
EndSub
Example:
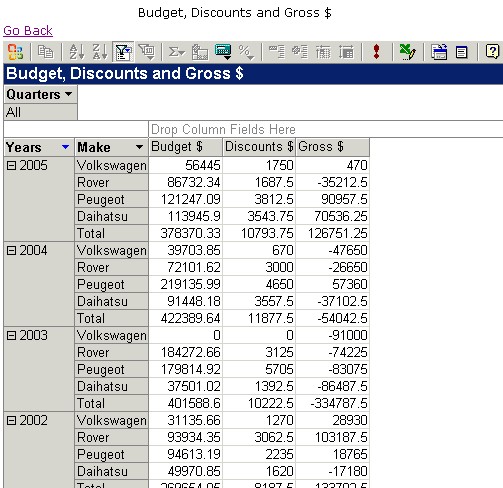
Verify the GXBIProperty SDT to know the options supported by each method.
Applying a specific Format to a query
If you want to apply a specific format to a query result (XmlAbstract) you can use the XSLTApply function (available in GeneXus 9.0) to transform the query result in XML format to HTML format in the client.
Based on a query result, let's say that in the &SpecificFormat variable you must call the associated function and then load the associated result (&HtmlFormat) in a TextBlock (with HTML format).
Event 'ExecuteQueryWithXSLT'
&ws.GetQueryData(/* Parameters */)
If &status.Error = 0
// OK
If &ApplyXSL = 'Y' // Flag to use the transformation
&HtmlFormat = XSLTApply( &specificformat, &XSLTFile)
endif
QueryResult.Caption = &HtmlFormat
Else
&msg = &status.ErrorDescription
Do 'MsgError'
EndIf
EndEvent