Consider the following Transaction object defined as a Business Component:
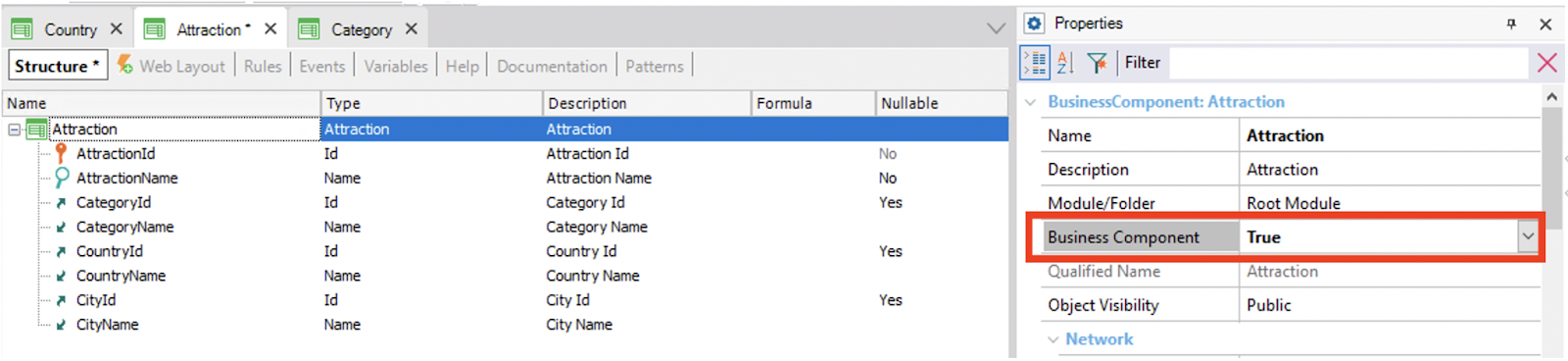
For all the following examples, you have to define an &Attraction variable based on the Attraction data type in a certain object (for example, in a Web Panel object, Panel object, or Procedure object). After that, you can code the following samples in the corresponding object section (Web Panel object Events, Panel object Events, Procedure object Source, etc.).
Suppose you want to insert a new attraction.
1.1) To do so, you can use the Business Component Save method as shown below:
&Attraction.AttractionName = "Eiffel Tower"
&Attraction.CountryId = 2 //France
&Attraction.CityId = 1 //Paris
&Attraction.Save()
If &Attraction.Success()
commit
msg("The data has been added")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
Notes
- The CategoryId was omitted, but since that foreign key allows nulls, the record will be inserted without failure.
- The AttractionId has its Autonumber property set to True, so it will be autonumbered by the database.
- After executing the Save() method, &Attraction.Mode() is set to Update ("UPD") and all attributes are instantiated.
1.2) You can use the Business Component Insert method as shown below:
&Attraction.AttractionName = "Eiffel Tower"
&Attraction.CategoryId = 2 //Monument
&Attraction.CountryId = 2 //France
&Attraction.CityId = 1 //Paris
&Attraction.Insert()
If &Attraction.Success()
Commit
msg("The data has been added")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
Read Differences between the Save method and the Insert and Update methods.
1.3) The following sample is almost the same as the previous one. The only difference is that the result of applying the Insert method is directly evaluated with an if sentence (so, the Success method is not used):
&Attraction.AttractionName = "Eiffel Tower"
&Attraction.CategoryId = 2 //Monument
&Attraction.CountryId = 2 //France
&Attraction.CityId = 1 //Paris
If &Attraction.Insert()
Commit
msg("The data has been added")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
1.4) You can use a Data Provider object.
To do so, create a Data Provider (for example, named DPOneAttraction). Drag the Attraction Transaction from the KB Explorer to the Data Provider Source and fill in the data as shown:
Attraction
{
AttractionName = "Eiffel Tower"
CategoryId = 2
CountryId = 2
CityId = 1
}
Next, call the Data Provider in the context you are positioned (for example, in the Events section of a Panel object or Web Panel object):
Event 'InsertAttraction'
&Attraction = DPOneAttraction()
If &Attraction.Insert()
commit
msg("The update has been successfully completed")
Else
rollback
msg("Unable to insert")
Endif
Endevent
1.5) Now, suppose you want to insert several attractions. To do so, you can define a Data Provider (for example, named DPSeveralAttractions).
First, drag the Attraction Transaction from the KB Explorer to the Data Provider Source and fill in the data, as shown below:
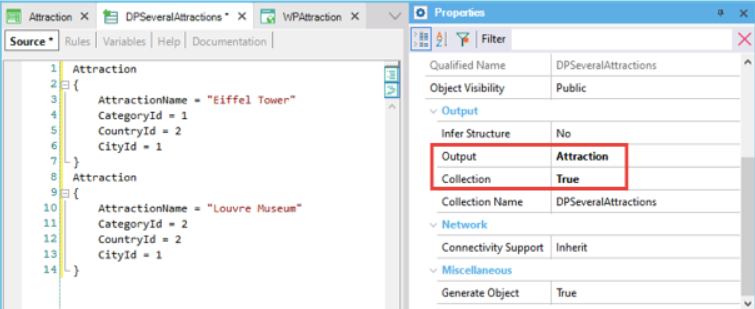
Note that the Data Provider Output property was filled automatically (with “Attraction”) because you dragged the Attraction Transaction to the source.
To indicate that you want to return several attractions, set the Collection property to True.
After defining the Data Provider, define a variable (for example, named &Attractions) in an object (for example, in a Panel, Web Panel, or Procedure) and set it as a collection by selecting the “Is Collection” checkbox, as shown in the image below:
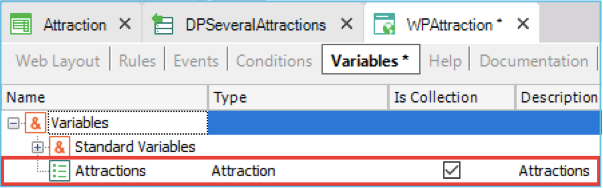
Next, call the Data Provider in the context you are positioned (for example, in the Events section of a Panel object or Web Panel object) and complete the code as shown below:
Event 'InsertAttractions'
&Attractions = DPSeveralAttractions()
If &Attractions.Insert()
commit
msg("The data has been added")
Else
rollback
msg("Unable to insert")
Endif
Endevent
Note that you load the attractions data inside the Data Provider and then call the Data Provider that returns the data in a variable set as a collection. Finally, the data is inserted into the database.
1.6) Now, consider the following two-level Transaction set as Business Component:
Attraction
{
AttractionId* (Autonumber property = Yes)
AttractionName
CategoryId
CategoryName
CountryId
CountryName
CityId
CityName
Ticket
{
AttractionTicketId*
AttractionTicketDescription
AttractionTicketPrice
}
}
Its first level exactly matches the Attraction Transaction Structure used for the above examples. Also, it contains a nested level.
Suppose you want to insert a new attraction with two lines (two tickets).
To do so, create a Data Provider (for example, named DPAttractionWithTickets).
Next, drag the Attraction Transaction from the KB Explorer to the Data Provider Source and fill in the data as shown:
Attraction
{
AttractionName = "Eiffel Tower"
CategoryId = 2
CountryId = 2
CityId = 1
Ticket
{
AttractionTicketId = 1
AttractionTicketDescription = "Popular"
AttractionTicketPrice = 100
}
Ticket
{
AttractionTicketId = 2
AttractionTicketDescription = "Vip"
AttractionTicketPrice = 300
}
}
The Data Provider Output property will be filled automatically with the “Attraction” value because you dragged the Attraction Transaction to its source.
After defining the Data Provider, define a variable (for example, named &AttractionWithTickets) in an object (for example, in a Panel, Web Panel, or Procedure) based on the Attraction Business Component data type. Finally, call the Data Provider in the context you are positioned (for example, in the Events section of a Panel object or Web Panel object) and complete the code as shown below:
Event 'InsertAttractionWithTickets'
&AttractionWithTickets = DPAttractionWithTickets()
If &AttractionWithTickets.Insert()
commit
msg("The data has been added")
Else
rollback
msg("Unable to insert")
Endif
Endevent
Note that you load the attraction with two tickets' data inside the Data Provider and then call the Data Provider that returns the data in a variable. Finally, the data is inserted into the database.
Suppose you need to update certain attraction data (for example, its category).
2.1) You can use the Business Component Save method as shown in the code below:
&Attraction.Load(1)
&Attraction.CategoryId = 1 //Monument
&Customer.Save()
If &Attraction.Success()
commit
msg("The data has been updated")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
2.2) You can use the Business Component Update method as shown below:
&Attraction = new()
&Attraction.AttractionId = 20
&Attraction.CategoryId = 2 //Monument
If &Attraction.Update()
Commit
msg("The update has been successfully completed")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
Read Differences between the Save method and the Insert and Update methods.
2.3) You can use a Data Provider object.
To do so, create a Data Provider (for example, named DPOneAttraction). Drag the Attraction Transaction from the KB Explorer to the Data Provider Source and fill in the data as shown:
Attraction
{
AttractionId = 1
CategoryId = 1
}
After that, call the Data Provider in the context you are positioned (for example, in the Events section of a Panel object or Web Panel object):
Event 'UpdateAttraction'
&Attraction = DPOneAttraction()
If &Attraction.Update()
commit
msg("The update has been successfully completed")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
Endevent
2.4) Now, suppose you want to update several attractions. To do so, you can define a Data Provider (for example, named DPSeveralAttractions).
Drag the Attraction Transaction from the KB Explorer to the Data Provider Source and fill in the data as shown below:
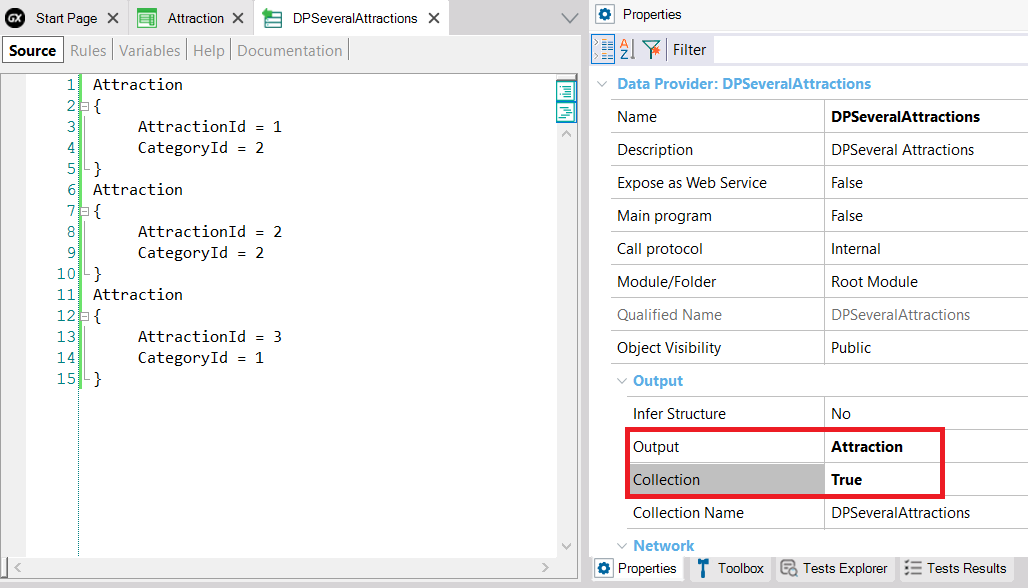
Note that the Data Provider Output property was filled automatically (with “Attraction”) because you dragged the Attraction Transaction to the source.
To indicate that you want to return several attractions, set the Collection property to True.
After defining the Data Provider, define a variable (for example, named &Attractions) in an object (for example, in a Panel, Web Panel, or Procedure) and set it as a collection by selecting the “Is Collection” checkbox, as shown in the image below:
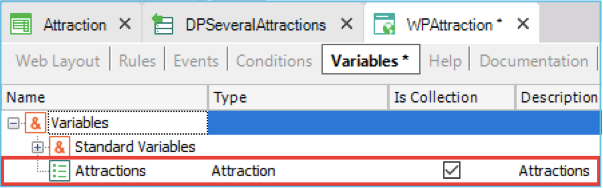
Next, call the Data Provider in the context you are positioned (for example, in the Events section of a Panel object or Web Panel object) and complete the code as shown below:
Event 'UpdateAttractions'
&Attractions = DPSeveralAttractions()
If &Attractions.Update()
commit
msg("The data has been updated")
Else
rollback
msg("Unable to update")
Endif
Endevent
Note that you load the attractions data inside the Data Provider and then call the Data Provider that returns the data in a variable set as a collection. Finally, the data is updated in the database.
3.1) To delete an attraction—for example, the Attraction with ID 1—use the Business Component Delete method as shown below:
&Attraction.Load(1)
&Attraction.Delete()
If &Attraction.success()
commit
msg("The update has been successfully completed")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
3.2) To delete several attractions—for example, all the monuments (attractions with CategoryId=2)—scan the desired attractions using the For each command. Next, inside the loop load each attraction and delete it as shown:
For each Attraction
where CategoryId = 2
&Attraction.Load(AttractionId)
&Attraction.Delete()
If &Attraction.success()
commit
Else
rollback
Endif
Endfor
Read the article titled Business Component InsertOrUpdate method that describes several examples.
Read the article titled Business Component Add method that describes an example to solve it.
Consider the following two-level Transaction set as Business Component:
Attraction
{
AttractionId*
AttractionName
CategoryId
CategoryName
CountryId
CountryName
CityId
CityName
Ticket
{
AttractionTicketId*
AttractionTicketDescription
AttractionTicketPrice
}
}
6.1) Suppose you need to update for the AttractionId = 6 its AttractionTicketId=3 with a different price.
Read the article titled Business Component GetByKey method article that solves this case.
6.2) Considering the same two-level Transaction set as Business Component, suppose that for AttractionId = 6 you need to update the price of the ticket with description="Without tour guide".
To achieve this, the code is as follows:
&Attraction.Load(6)
For &Ticket in &Attraction.Ticket
If &Ticket.AttractionTicketDescription="Without tour guide"
&Ticket.AttractionTicketPrice=100
Endif
Endfor
&Attraction.Save()
If &Attraction.success()
commit
msg("The update has been successfully completed")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
Consider the following two-level Transaction set as Business Component:
Attraction
{
AttractionId*
AttractionName
CategoryId
CategoryName
CountryId
CountryName
CityId
CityName
Ticket
{
AttractionTicketId*
AttractionTicketDescription
AttractionTicketPrice
}
}
7.1) Suppose that for AttractionId = 6 you need to delete the line with AttractionTicketId=3.
Read the article titled Business Component RemoveByKey method that solves this case.
7.2) Considering the same two-level Transaction set as Business Component, suppose you need to delete the first Ticket of the AttractionId = 6, regardless of the AttractionTicketId value.
To achieve this, the code is as follows:
&Attraction.Load(6)
&Attraction.Ticket.Remove(1)
&Attraction.Save()
If &Attraction.success()
commit
msg("The line deletion has been successfully completed")
Else
rollback
msg(&Attraction.GetMessages().ToJson())
Endif
Read the article titled Business Component - Publication as Web Service that contains examples.