Through the KB Explorer you can go to the GeneXus > Common > Configuration module and find the domain named StorageProviderType.
By defining variables based on this domain, you are able to use methods to manage the application's global data (any type of files) in an external storage. The external storage can be Amazon, Azure, Google, or IBM Cloud (the possible external storages are the same as the Storage Provider property values).
Saves a file to the external storage, and returns TRUE if the operation was successful.
You can use the OutUploadedFile parameter if you want to get a reference (File data type) of the file in the external storage. Use GetURI() to get the URI of the file in the storage.
Saves the file as private in the external storage and returns TRUE if the operation was successful.
The file is signed and uploaded to the bucket. The certificate used to sign the file is generated by the provider.
Return value |
Boolean |
Parameters |
FileFullPath:Character, [StorageObjectFullName:Character], [OutUploadedFile:File], [OutMessages:Messages] |
You can use the OutUploadedFile parameter to get a reference (File data type) of the file in the external storage. Use GetURI() to get the URI of the file in the storage.
Gets a reference (File data type) of the file in the external storage. Returns TRUE if the operation was successful.
Return value |
Boolean |
Parameters |
StorageObjectFullName:Character, OutExternalFile:File, [OutMessages:Messages] |
GetPrivate
Gets a reference (File data type) of the file in the external storage. Returns TRUE if the operation was successful.
Return value |
Boolean |
Parameters |
StorageObjectFullName:Character, OutExternalFile:File, ExpirationMinutes:Numeric, [OutMessages:Messages] |
OutExternalFile is the file retrieved from the bucket and contains the URL.
The URL obtained is valid only for a period of time, determined by the ExpirationMinutes parameter.
Downloads the external file to the application server and saves it locally. Returns TRUE if the operation was successful.
Return value |
Boolean |
Parameters |
StorageObjectFullName:Character, OutLocalFile:File, [OutMessages:Messages] |
With the OutLocalFile you can define where the external file is going to be saved after being downloaded.
(Only applies to Azure Storage Provider)
Downloads the private external file to the application server and saves it locally. Returns TRUE if the operation was successful.
Return value |
Boolean |
Parameters |
StorageObjectFullName:Character, OutLocalFile:File, [OutMessages:Messages] |
With the OutLocalFile you can define where the external file is going to be saved after being downloaded.
Gets a Directory in the external storage (the cloud).
Return value |
Boolean |
Parameters |
StorageDirectoryFullName:Character, OutExteralDirectory:Directory, [OutMessages:Messages] |
In Amazon, the format is <bucketname>: Directory
For example: genexuss3test:\petsFolder\
- The StorageObjectFullName is the full name that the file has in the bucket (including folders and extension).
- The Messages object is a collection of Messages.Message with the following format:
- Id: The error code returned by the Storage Provider (Amazon S3, Google, etc)
- Type: (0 = Warning,1 = Error)
- Description: The error description returned by the Storage Provider.
When there is no error, the &Messages collection is empty.
Consider a scenario where you need to upload files to the external storage manually. Note that the file to be uploaded to the external storage has been uploaded previously to the application server.
First, define a &Storage variable, of StorageProvider data type.
The file to be saved to the external storage is originally located on the server's file system, so use the File data type to get its absolute path.
The Upload method of the Storage Provider API allows you to give a full name to the file being uploaded, including folders and extension.
&Storage = new() //This line of code creates a new instance of the external storage configured in the KB
&File.Source ="catToUpload.jpg" //&File is of File data type.
&FileFullPath = &File.GetAbsoluteName()
&StorageObjectFullName = "petsFolder/cat.jpg"
if ( &Storage.Upload(&FileFullPath, &StorageObjectFullName, &UploadedFile, &Messages) )
&URL = &UploadedFile.GetURI() //&URL of the uploaded file
else
for &Message in &Messages
msg(&Message.Description, status)
endfor
endif
In this sample, given that the bucket name is genexuss3test, the folder petsFolder is created under that bucket and contains the file uploaded.
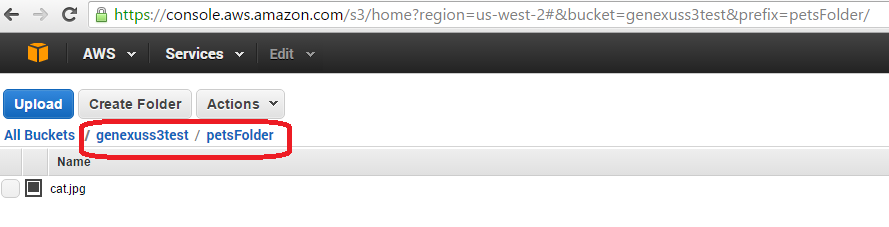
Here you get the URI of the file located in the external storage. &ExternalFile is of File data type.
&StorageObjectFullName = "petsFolder/cat.jpg"
if ( &Storage.Get(&StorageObjectFullName, &ExternalFile, &Messages) )
msg(format("The external URI of the image is : %1", &ExternalFile.GetURI()), status)
else
for &Message in &Messages
msg(&Message.Description, status)
endfor
endif
It returns: https://genexuss3test.s3.amazonaws.com/petsFolder/cat.jpg
This sample shows you the code to download the external file to the server's file system. &LocalFile is of File Data type.
&StorageObjectFullName = "petsFolder/cat.jpg"
&LocalFile.Source = "catDownloadFromStorage.jpg"
if ( &Storage.Download(&StorageObjectFullName, &LocalFile, &Messages) )
msg(format("Image downloaded to : %1", &LocalFile.GetAbsoluteName()), status)
else
for &Message in &Messages
msg(&Message.Description, status)
endfor
endif
Here, you remove all the files from the external storage folder. &ExternalDirectory is of Directory data type.
Note that the file is treated as an external file here.
&DirectoryFullName = "petsFolder"
if ( &Storage.GetDirectory(&DirectoryFullName, &ExternalDirectory, &Messages) )
for &ExternalFile in &ExternalDirectory.GetFiles()
&ExternalFile.Delete()
endfor
else
msg(format("The directory %1 couldn't be obtained", &DirectoryFullName))
for &Message in &Messages
msg(&Message.Description, status)
endfor
endif
&StorageObjectFullName = "folder/catToUpload.jpg"
&found = &StorageProvider.Get(&StorageObjectFullName, &File, &outMessages)
If &found
&File.Delete()
Else
Log.Error(!"File not Found")
EndIf
&Storage = new()
&File.Source = "catToUpload.jpg"
&FileFullPath = &File.GetAbsoluteName()
&StorageObjectFullName = "Private/cat1.jpg"
if (&Storage.UploadPrivate(&FileFullPath, &StorageObjectFullName) )
msg("File has been successfuly uploaded", status)
else
for &Message in &Messages
msg(&Message.Description, status)
endfor
endif
&Storage.GetPrivate(&StorageObjectFullName,&UploadedFile,11)
msg(&UploadedFile.GetURI(), status) //URL is valid during 11 minutes
Download the complete sample from Storage provider API sample.
See Configuration.ExternalStorage External Object.
The Storage Provider API is available for Java and .NET Generators and all Storage providers supported by the Storage Provider property.
Exception: UploadPrivate and GetPrivate are available only for .NET Generator and Amazon S3 since GeneXus 15 Upgrade 3. They are available for .NET Generator and Java Generators for Amazon S3 and Microsoft Azure since GeneXus 15 Upgrade 7. These methods will be available for the rest of platforms in the upcoming upgrades.